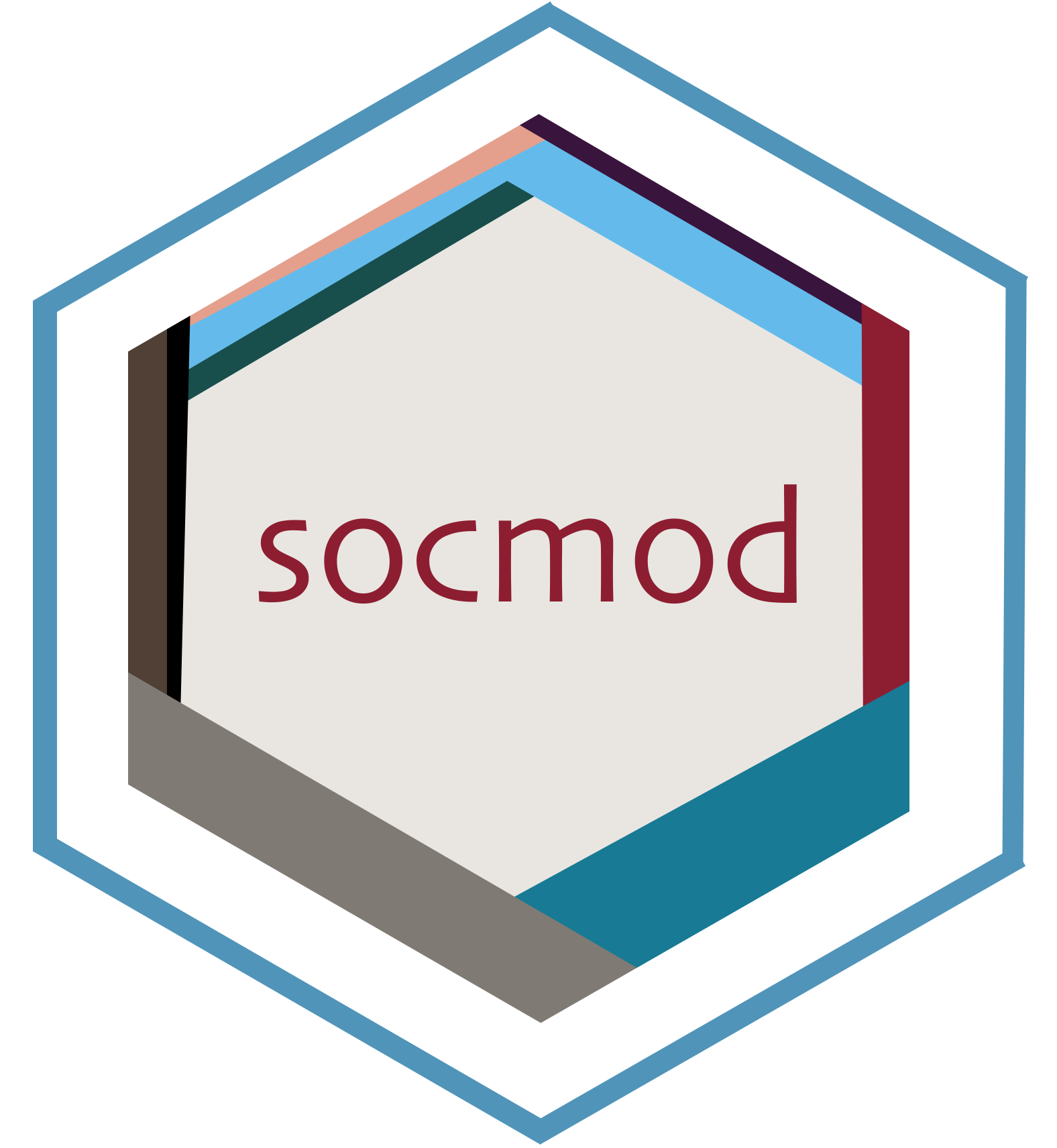
Initialize agents with adaptive and legacy behaviors
initialize_agents.Rd
Assigns behaviors and fitness values to agents in an AgentBasedModel. Can initialize by proportion or fixed count of adaptive agents.
Usage
initialize_agents(
model,
initial_prevalence = 0.1,
adaptive_behavior = "Adaptive",
adaptive_fitness = 1.2,
legacy_behavior = "Legacy",
legacy_fitness = 1
)
Arguments
- model
An
AgentBasedModel
instance.- initial_prevalence
A proportion (0–1) or count of agents starting with the adaptive behavior.
- adaptive_behavior
Name of the adaptive behavior (default: "Adaptive").
- adaptive_fitness
Fitness value for adaptive behavior (default: 1.2).
- legacy_behavior
Name of the legacy behavior (default: "Legacy").
- legacy_fitness
Fitness value for legacy behavior (default: 1.0).
Examples
# Create a model with 20 agents, 25% with adaptive behavior
abm <-
make_abm(n_agents = 20) |> initialize_agents(initial_prevalence = 0.25)
# Count how many agents do each behavior
table(purrr::map_chr(abm$agents, ~ .x$get_behavior()))
#>
#> Adaptive Legacy
#> 5 15
# Summarize fitness values by behavior
tibble::tibble(
behavior = purrr::map_chr(abm$agents, ~ .x$get_behavior()),
fitness = purrr::map_dbl(abm$agents, ~ .x$get_fitness())
) |>
dplyr::group_by(behavior) |>
dplyr::summarise(count = dplyr::n(),
mean_fitness = mean(fitness),
.groups = "drop")
#> # A tibble: 2 × 3
#> behavior count mean_fitness
#> <chr> <int> <dbl>
#> 1 Adaptive 5 1.2
#> 2 Legacy 15 1